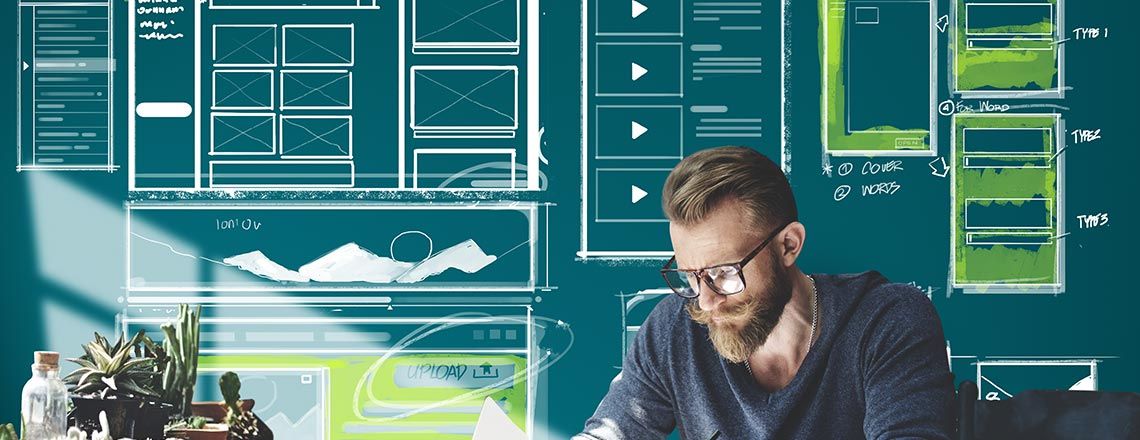
The Node.js runtime environment features everything required to execute JavaScript programs, so it’s important to have a basic understanding of JavaScript before getting started.
Evolution of Node.js
When JavaScript was first created, it could only run in the browser. The developers created Node.js to enable JavaScript to run in the form of a standalone application that allowed it to do more than just add interactivity to a website, making it more similar to Python and other scripting languages.
Both Node.js and JavaScript run using the V8 scripting runtime engine, which converts the code into machine code, a low-level code that computers can run without any need to interpret it.
Defining Node.js
Node.js uses an event-driven, non-blocking I/O model, making it efficient and lightweight. Its npm package ecosystem is the largest in the world of open-source libraries.
A Closer Look at the Node.js I/O Model
I/O stands for input/output and can refer to any type of the two, which may include making HTTP requests to APIs or reading and writing local files. However, most I/O systems take time to perform these processes, which can block other functions that require completion.
Blocking vs. Non-Blocking I/O
The Node.js I/O model is non-blocking, but what does that mean?
For systems that operate on a blocking model, only one action can be completed at a time. For example, you might request details about two users from a back end database to have them print on the screen; the blocking method would require the system to find and print the data for the first use before starting the process for the second user. Additionally, the web server would need to begin new threads for each user, and since JavaScript is single-threaded, it would not do well with multi-threaded tasks.
Non-blocking requests resolve this issue by starting the data request for the second user before the request response for the first user’s data appears, making it possible to initiate both requests simultaneously, eliminating the need for multi-threading.
How Node.js Handles File Requests
Web servers frequently need to open files before giving the content to the client, so it’s important to understand how Node.js handles file requests.
With PHP or ASP, the file request is sent to the computer’s file system. The content is returned to the client once the file system opens and reads the contents, at which point the ASP or PHP can handle the next request.
By contrast, Node.js sends the task directly to the file system so it is ready to handle your request. Once the system opens and reads the file, it returns the content. Essentially, Node.js eliminates the wait and moves on to the next request. This non-blocking, asynchronous and single-threaded programming is also very efficient for memory.
Key Features of Node.js
There are a variety of reasons why software architects choose to use Node.js. We’ll explore its more important features below.
Event-driven and Asynchronous
Every API in the Node.js library is asynchronous or non-blocking. As such, a server based on Node.js will never wait for the API to return data.
Speed
Being built on the Chrome V8 JavaScript Engine helps make code execution with Node.js’s very fast.
Single-Threaded With High Scalability
When we say that Node.js is single-threaded, we mean that it uses a single-threaded model that includes event looping, making it possible for a program to serve more requests than a traditional server. The Event mechanism assists the server with non-blocking responses, allowing for high scalability, whereas traditional servers provide limited threads for handling requests.
To better understand event looping, take a second to see how JavaScript Event Loop functions. Push main ( ) on the call stack, then push console.log ( ) on it. This will run immediately, getting popped. Then push setTimeout (2000) onto the stack. This is a Node.js API that will register an event-callback pair when called. The function of the callback will occur after the event waits 2,000 milliseconds.
Once the event is registered in the APIs, the setTimeout (2000) is popped from its call stack. Now a setTimeout (0) is registered similarly, meaning that there are two Node.js APIs waiting for execution. setTimeout (0) will wait 0 seconds before moving to the queue for the callback, along with setTimeout (2000).
After moving to the callback queue, the functions wait for an empty call stack since only a single statement can execute at once. That occurs thanks to the event loop. Finally, the final console.log ( ) will run, with the main ( ) getting popped from its call stack. At this point, the event loop notices the call stack is empty, but the callback queue is not. It then moves the callbacks to the call stack so that they can be executed, doing so first in, first out.
Licensing
Node.js is released under the MIT license, making it easy to access and use.
No Buffering
Node.js applications output data in chunks, so you never have to deal with buffering.
Understanding Node.js Files
When you create a Node.js file, you will include tasks that are executed for certain events, for example, someone attempting to access a server’s port. All Node.js files use the .js extension and must be initiated on the server. If not, they will not have an effect.
Node.js Package Manager
The Node.js package manager (npm) is a set of community-built libraries that can resolve most of the generic problems you might encounter. This easy-to-use, default tool features a range of packages that can improve the speed and efficiency of development.
Using Require
Require is a key element that performs a few key tasks like loading modules bundled with file systems such as Node.js or HTTP from your API. The function accepts a parameter of “path” and will return module.exports. It also loads third-party libraries installed from npms and, as its name suggests, allows you to require the use of your own files and modularization of the project.
Understanding Node.js Modules
Node.js modules are reusable blocks of code whose existence must not accidentally impact any other code. The system comes with built-in modules, and once you have some practice, you can write them yourself for use in applications.
What You Can Do With Node.js
Node.js can also be used to create dynamic page content and can perform the following actions to server files: creating, opening, reading, writing, deleting and closing. It can also collect form data or add, modify or delete data in a database.
Node.js can be used for I/O bound applications, data streaming applications, DIRT (data-intensive real-time applications), single-page applications and JSON APIs-based applications, as well as CHAT and APIs on object DBs. However, it is not ideal for CPU-intensive applications.
Because of its extensive capabilities, Node.js is used by developers and major companies including Uber, Wikipins, PayPal, Microsoft, GoDaddy, General Electric, Yammer and Yahoo!.
How to Write “Hello World”
Using the classic example, let’s write “Hello World” with Node.js. Start by creating a file with a .js extension and add the following code:
console.log(“Hello World!”);
Now open up your Node.js terminal. Go to the directory and change it to whichever folder you saved your new .js file in. Run node __.js where __ is the name of your file.
Conclusion
If your interest in Node.js is piqued, consider enrolling in a bootcamp to gain a deeper understanding. With the right knowledge, you can enhance your programming using Node.js.
*Please note, these articles are for educational purposes and the topics covered may not be representative of the curriculum covered in our boot camp. Explore our curriculum to see what you’ll learn in our program.
Get Program Info
Ready to learn more about Berkeley Coding Boot Camp in San Francisco? Contact an admissions advisor at (510) 306-1218.