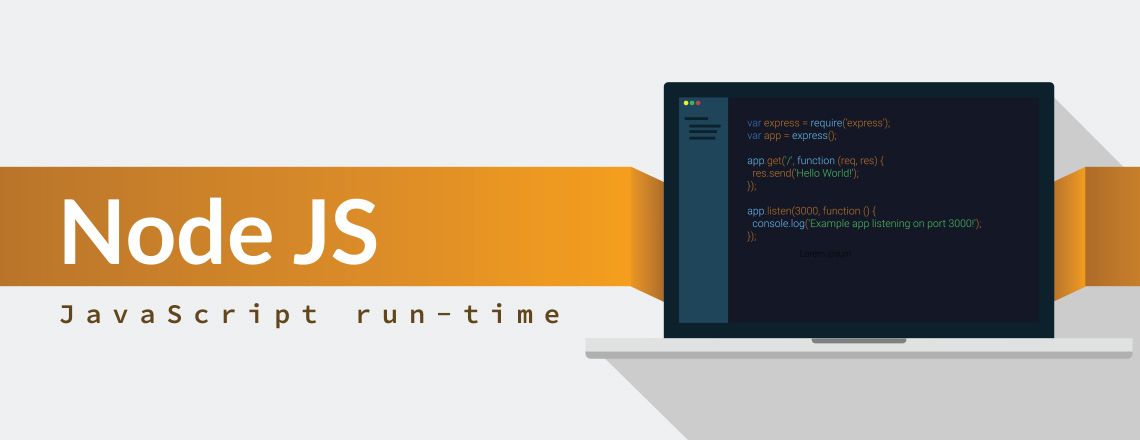
Node Redis is a quick, efficient in-memory key-value store that also serves as a data structure, and can change the way you design your apps. It is a quick, efficient and powerful development tool that supports a wide array of data structures including hashes, strings, sets, lists and more, giving app developers a much higher degree of flexibility.
In this tutorial, you will explore some of the ways Node.js and Redis interact using the node_redis library, as well as learn how to build and speed up a starter application. You will also see how apps can be used to seamlessly retrieve data from APIs and display it to users.
Starting Out: Installing Node Redis
node_redis is Node.js’s Redis client. Install it using npm by running the command npm install redis. After you have successfully installed the node_redis module, create a simple file called app.js to demonstrate how it connects Node.js to Redis.
Redis.createClient() uses 127.0.0.1 and 639 as its default hostname and port. If you have another host or port, you can add them like so:
You can then perform an action after the connection is established and listen for connect events. For example:
The following is inserted into app.js:
Once finished, ensure that the Redis server is up, then type node app into the terminal to run the app.
How to Store Key-Value Pairs
Store key-value pairs using the storage Redis makes available; every Redis command is exposed as a different function on the client object. So, if you want to store a simple string, use:
Either snippet will work for these purposes, as they both store a simple string called AngularJS against the key framework, each in its own way. The first snippet passes a variable number of arguments, whereas the second snippet passes an args array to the client.set() function. You also have the option of passing an optional callback, which will give you a notification once the operation finishes:
If for some reason an issue occurs and the operation fails, the err argument to the callback will show you the error. If you need to retrieve a key value, enter:
Retrieve keys stored in Redis by using client.get(), and access the value of a key by using the callback argument reply. If a key does not exist, you will see an empty reply value.
How to Store Hash
Storing simple values will rarely be enough to solve a problem. Many times, you will have to store hashes or objects in Redis. To store hashes, use the hmset() function:
This snippet stores the hash in Redis, which maps each technology into its own framework. So, the first argument to hmset() is the key’s name, and the following arguments are for key-value pairs. Hgetail() can be used to retrieve the key’s value. If you find the key, the second argument to the callback has the value (an object).
Redis offers no support for nested objects. Every property value in an object is coerced into strings before Redis stores it. You also have the option of storing objects in Redis using this syntax:
You can also pass an optional callback, so you know when the operation finishes. Functions (commands) can be called with lower and uppercase equivalents, so either client.hmset() or client.HMSET() are equivalent.
How to Store Lists
Use Redis lists to store a list of items with the following syntax:
This creates a list called frameworks and will push two elements into it, making the list’s length two. An args array has been passed to rpush here. The array’s first item represents the key’s name, while the rest of the items represent list elements. Use lpush() rather than rpush() to push elements left instead of right. To retrieve elements in the list, use the lrange() function:
You can get all the elements in a list anytime you need to by passing -1 as the third argument to lrange(). You need to pass the end index here if you are trying to get a subset of the list.
How to Store Sets
Unlike lists, sets do not allow for duplicates. To use one, modify the previous snippet to use a set in place of a list, like this:
The sadd() function creates a new set that has the specified elements and a length of three. Retrieve members of the set by using the smembers() function:
Use this snippet to retrieve all the set’s members, however the order is not preserved when you retrieve the members.
Not only can you store lists, strings, hashes and sets, but you also can store hyperLogLogs, sorted sets and more by using Node Redis. Check the official Node Redis documentation for a complete list of data structures and commands available to you.
There are a few additional important operations that node_redis supports.
Checking if Keys Exist
The process to check the existence of keys before proceeding is simple: use the exists() function like so:
How to Delete and Expire Keys
It is also quite simple to delete and expire keys, or clear keys and reinitialize them. When this happens, use the del command to clear the keys.
You also have the option of giving an existing key an expiration time. This snippet will assign a 30-second expiration time to key1:
Decrementing and Incrementing
You can also use Node Redis to decrement and increment keys. To increment a key, use the incr() function:
Keep in mind that the incr() function uses increments of 1 for key values. If you want to change the incr() to increment by a different amount, use the incrby() function. You can decrement keys by using the decr() and decrby() functions.
Next Steps in Mastering Node Redis
If you are working in a tech hub like San Francisco, Node.js classes can help you master the intricacies of using Node Redis and help you work out difficulties with real-time support and learn more thoroughly through joint projects with peers.
*Please note, these articles are for educational purposes and the topics covered may not be representative of the curriculum covered in our boot camp. Explore our curriculum to see what you’ll learn in our program.
Get Program Info
Ready to learn more about Berkeley Coding Boot Camp in San Francisco? Contact an admissions advisor at (510) 306-1218.