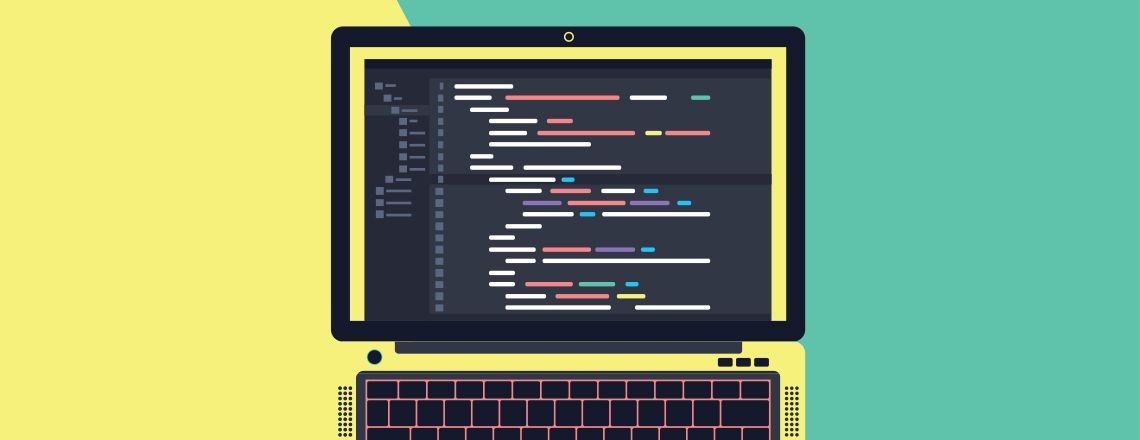
No programmer likes a slog. Sure, it might be fun to build a project from scratch now and again — but when you’re up against project deadlines, you don’t have time to fiddle around with the basics. That’s why you use Django.
Django is a free, open-source Python framework designed to take the hassle and fuss out of back end programming. With it, developers can create complex, secure and scalable apps quickly. Every back end or full stack coder who appreciates efficiency and clean code can benefit from having Django in their tool box.
But where should you start? In this article, we’ll walk you through everything you’ll need to know before you learn Django, from the framework’s history to its real-world applications.
What Is Django?
Django is a high-level and open-source framework that helps Python developers write pragmatic, functional code quickly. It can be used to construct nearly any type of site a coder could dream up — from blogs to wikis to social media networks and beyond. It’s compatible with all client-side frameworks and is well-known for its functionality.
Django was made with comprehensiveness in mind. As the framework’s creators explain on its website, Django’s intent is to “take care of much of the hassle of Web development, so you can focus on writing your app without needing to reinvent the wheel.”
As a result, developers can access any and all tools they would need to develop top-notch projects. Django’s framework is pre-engineered to uphold security best practices and offers several database options and templating engines. Developers who use Django enjoy unparalleled flexibility as well as unusually comprehensive support. Plus, because the platform is open-source, all of its documentation is readily available online.
Why Use Django?
Django’s capacity to accelerate and improve the web development process speaks for itself. Programmers who use the framework can readily design, launch and maintain functional sites without getting weighed down by the tedious development elements. Plus, sites built with Django benefit from being notably secure, scalable, versatile, portable and maintainable.
In short, using Django will make you a more effective and efficient developer.
Skills covered in these tutorials:
- URL Mapping
- Form Validation
- Population Scripts
- Regular Expressions
- Modules & Packages
- Decorators
Introduction to Django
Django is an undeniably valuable tool in any developer’s skill set — but getting started with it can be challenging. In the below sections, we’ll share everything you need to know before you learn Django, as well as the framework’s history, structure and setup.
What You Need to Know Before Getting Started
First and foremost, you need to know Python. Django’s framework is based around Python programming; if you don’t know the language, you won’t be able to use the tool.
You should also have a basic understanding of coding fundamentals. While the Django framework provides a simplified website-building experience, users must understand web development principles to use the framework effectively.
Aspiring Django users should also have a grasp on the framework’s somewhat-opinionated philosophy so they can decide if it meshes with their coding preferences.
Here’s a quick rundown on what the term means in a programming context. An opinionated programming framework has pre-built assumptions on what constitutes the “correct” way to build a functional website. These often provide copious documentation and pre-established solutions to common coding problems. However, opinionated platforms tend to be relatively inflexible and may restrict a developer’s ability to use components or approaches that aren’t already defined in its conventions.
An un-opinionated framework, in contrast, eschews support in favor of flexibility. Developers can use whatever tools or approaches they please, so long as they don’t mind personally importing them.
A somewhat-opinionated philosophy falls somewhere in between the two. As a platform that embraces this ideology, Django offers a comprehensive set of components and a few preferred coding approaches. However, it does not limit coders to these structures. Django users can add new components as they please and use unconventional coding solutions as readily as pre-packaged ones.
Once you have a firm grasp of web development principles, Python coding and the framework’s philosophy, you can start to learn Django.
Interested in learning more about skills you need to become a web developer?
Get Program Info
Where Does Django Come From?
Django was first conceptualized in 2003 by Adrian Holovaty and Simon Willison. The two were prolific website builders who realized, after a time, that they didn’t need to continually build their sites from scratch. Instead, they could reuse some of the code and designs they had already created — and thus significantly expedite the web development process.
It took two years, but the reusable code the pair identified was eventually packaged and formally released as an open-source web development framework named Django in 2005.
In the decade-plus since Django’s debut, the project has expanded into a massive, collaborative endeavor that includes thousands of contributors and users. With each new release of the framework (including the recent 3.1 version released in 2020), Django improves functionality, addresses more common issues and increases feature support.
Django has aided in the development of several high-profile sites, including those for National Geographic, Pinterest and Instagram. While there are other frameworks that developers can use — and it is, of course, still possible to build sites and apps from scratch — Django remains one of the most efficient ways to develop high-performing sites quickly.
Structure of a Django Website
Sites and applications built using Django utilize a directory structure; in other words, they display system files in a hierarchical tree. There is room for some customization, but the default structure consists of certain files.
Let’s go over a quick Django tutorial for beginners!
manage.py
This file serves as a command-line utility — i.e., a tool that allows developers to give text-based instructions. Manage.py assists developers in testing, debugging and deploying their web applications. It also includes code used to run the application’s web server and create the website’s underlying database. This folder contains several key commands, which are bulleted below.
- runserver: As the name suggests, this command runs the server for a web application.
- migration: This command can create a database and “migrate,” or make changes to a database.
- makemigration: Your database will not reflect any migrations until you enact them with makemigration. This command applies prospective changes to your database.
my_project
The my_project package houses the configuration files for your project settings. These include:
- _init_.py — This file is (and should remain!) empty. Its purpose is to inform the Python interpreter that the directory is a package. This is standard among Python packages.
- settings.py — This file contains installed applications and all information pertaining to middleware or software that facilitates communication between the operating system and its applications. It also houses templates and databases.
- urls.py — Here, you can expect to find all URLs relating to your project. These include URLs for images, pages and applications. This file will process any relevant URLs and is generally used to connect the project with related web-apps.
- wsgi.py — The Web Server Gateway Interface (WSGI) specification outlines the standard interface that connects web servers with Python frameworks and applications. This file allows you to deploy your applications on a server. You will not need to make any changes to this file.
- asgi.py — Newer versions of Django also feature this file. ASGI, like WSGI, is a specification that defines interactions between Python-written applications and servers. ASGI can be considered the WSGI successor, as it provides more flexibility and freedom than the alternative. That said, both are still very much in use.
Understanding the files contained within the Django framework, as well as their functions, can be useful for programmers and web developers. Knowing what to expect from the framework will be crucial in ensuring that you can effectively use Django to build functional projects.
How to Set Up Your Development Environment
A development environment encompasses all of the tools and procedures you will need to develop, test and debug projects. Fortunately, the Django development environment is relatively easy to set up and use. Once you do, you’ll have access to several essential features, including:
- Python scripts
- A development web server
- A text editor or integrated development environment (IDE)
- A source control management tool
The Django setup process is remarkably convenient. Most operating systems — and all of those that can run Python — can support Django. This framework can also be installed from various sources, such as your computer’s package manager application or the Python Package Index (PyPi). Here’s a quick Django tutorial on how to set up your development environment:
- Ensure Python is installed
- Create a directory for your project
- Create a virtual environment
- Install Django to the virtual environment
- Verify Django installation and operation
- Create a new project
Following these simple steps will allow you to set up your development environment and utilize Django’s features.
Django Projects and Applications You Can Create
There are a near-infinite number of potential web and application projects developers can create with Django. Not sure where to start? Here’s a quick list of a few beginning to moderate challenges you can tackle.
- Design a login system template
- Create a simple chat function
- Build a site for securely storing passwords
- Develop a secure application for managing sensitive data
- Construct a “to-do” list app for managing tasks
- Design a web app for generating a resume from user-provided input
- Build a content management system
- Develop a secure, efficient site for hosting video chats
By tackling projects like those listed above, you can effectively build a substantial portfolio and acquire several functional templates for future coding endeavors.
That said, it’s also essential to identify when using Django is not ideal. It may be best to avoid Django in cases where:
- The application cannot be contained within a single codebase
- Your project has very simple demands and does not require any complex features
- You want to build a project from scratch
- Your knowledge of Python and/or Django is limited
If any of these caveats applies to you, you may want to either find an alternative coding framework or spend more time brushing up on your Python skills.
Who Needs to Learn Django and How Is It Used?
Django is a useful tool for any professional whose role requires back end or full stack coding skills. That said, there are a few jobs that outright require the framework. We’ve spotlighted a few below — check them out!
Python Developer
Python developers are programmers who, as you can probably guess from the title, code primarily in Python. Their exact responsibilities tend to vary across employers. However, these coders typically develop software and web applications, build tools, create applications and collaborate with back end or data analytic professionals. All Python developers can benefit from knowing Django, given that the framework was designed to accelerate and improve Python-based coding projects.
Application Developer
Application developers may also be referred to as software developers or architects. Put simply; application developers write and modify code to support software functionality and optimization. When working with web applications, these developers may use Django to expedite and simplify their code.
Back End Developer
Back end developers are responsible for building, managing and improving the data and logic structures that underlie a website or application. Their work complements the efforts of front end programmers who work on a website’s visible elements. Django is a framework designed specifically for all-side web development and so is an invaluable tool for any server-side professionals who enjoy coding in Python.
Front End Developer
While Django is not, strictly speaking, a necessity for front end developers, it can come in handy. These professionals are tasked with coding application interfaces and producing user-friendly designs. Django can be a useful tool for front end coders, given its fundamental ability to render HTML, easy form authentication and tight security.
Full Stack Developer
As the name suggests, full stack developers have command over the entire “stack” of web development technologies. They are equally comfortable designing front end interfaces as they are back end data structures. Their versatility makes them valuable in programming roles and allows them to code a variety of projects. Django, of course, supports web development and is thus a useful skill for any full stack professional.
How Long Does It Take to Learn Django?
As with any skill, learning how to master Django takes time and practice. If you already know Python and are familiar with technical concepts like terminology authentication, URL routing and API, you may be able to learn all you need to use Django in as little as two to three weeks.
Naturally, you should avoid rushing this process, as mastering Django requires that you understand the fundamental principles. Patience and consistent practice will pay off.
A cursory online search will provide you with a Django tutorial for beginners. That said, if you don’t learn well via independent study or want to upskill quickly, you may wish to enroll in formal, instructor-led Django courses.
What Other Skills/Languages Should I Learn?
You won’t be able to build a career in coding with Django alone. If you intend to become a full stack coder — or any other programming-adjacent professional — you should pick up a few core web development skills. These include (but are not limited to):
- SQL — Using Django often means working with databases, so learning SQL (a popular query language used for accessing, managing and manipulating database information) would be helpful.
- JavaScript — JavaScript is one of the most popular and commonly used programming languages in the coding sector. It’s not just a useful tool; it’s one that employers will likely expect you to have.
- HTML and CSS — If you plan to work in front end development, you need to know HTML and CSS. These technologies are fundamentally important to web development and underlie the vast majority of websites in operation today.
- Node.js and Bootstrap — Node.js and Bootstrap are both invaluable coding frameworks. The former is frequently used to code back end applications in JavaScript; the latter is a CSS tool that allows developers to create responsive, mobile-enabled websites. Both are invaluable to full stack coders and other programming professionals.
- Data Analysis — If you find that you enjoy working with databases and want to explore career options in that direction, you may want to study the principles and practices of data analysis.
Feeling overwhelmed? You don’t need to be. While all of these skills can technically be obtained through nose-to-the-grindstone independent study, that academic path can be a long and difficult one.
Learners who want to obtain job-ready skills quickly should consider more structured programs like Berkeley Coding Boot Camp and Berkeley Data Analytics Boot Camp. These intensive programs will arm beginning coders with the skill set and practical foundation they’ll need to land an entry-level job in the field of their choice.
Bootcamps are short and flexible; depending on whether they opt for a part- or full-time schedule, learners can finish upskilling in just three to six months. Programs are available in both in-person and virtual formats. Contact us today for more information on how we can springboard your career!
Django FAQs
What Is Django Used For?
Django is a free, open-source, collaborative web framework used to facilitate the development of secure, efficient, functional websites, web pages and web applications. You can use Django to develop website features that include but aren’t limited to chatbots, submission forms, video chat hosting and more. Using Django will ultimately save time and energy by providing you with standard coding procedures and templates to integrate into your projects. With these, you can avoid needing to build the foundations of your project from scratch.
Is Django Easy to Learn?
As a relatively simple framework, Django is not especially challenging to master, but it does require some prior knowledge of Python to utilize it effectively. Generally, most beginners can get a good introduction to Django within the span of a few weeks, though mastery requires additional time and practice.
Is Django a Programming Language?
Django is not a programming language; it is a coding framework built to be compatible with Python — which is a back end coding language.
Learning Django can be immensely beneficial for anyone aspiring to become a programmer or web developer. With Django, you can more effectively build functional, secure, versatile sites and applications that are both efficient and user-friendly. If you are interested in learning how to use this framework, consider reviewing online Django tutorials or enrolling in a coding bootcamp that covers the Django basics.
Navigate Django Articles
From basic knowledge to more advanced Django coding tutorials.
Get Program Info
Ready to learn more about Berkeley Data Analytics Boot Camp? Contact an admissions advisor at (510) 306-1218.