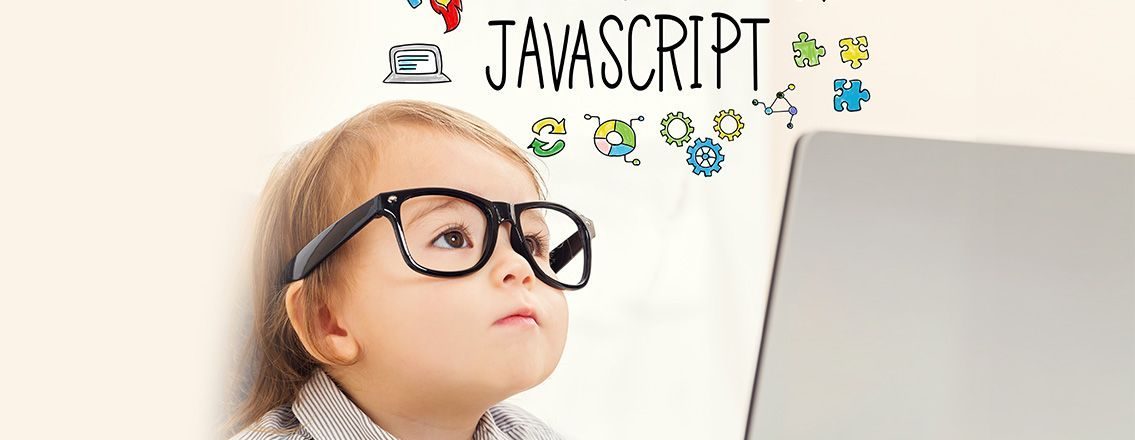
JavaScript Programming for the Absolute Beginner
If you want to make it as a professional coder, you’ll need to have JavaScript in your toolbox.
Today, JavaScript stands as one of the most popular and in-demand languages on the job market. Developers often use it to build websites, design games and craft applications, among other pursuits. The language is one of the fundamental skills you should have on your resume before entering the job pool.
But what should you know before you start to learn JavaScript? In this article, we’ll cover all the basics beginners need to know:
- Why Learn JavaScript?
- Introduction to JavaScript
- Uses of JavaScript
- JavaScript Careers
- Learning JavaScript
- JavaScript FAQs
- JavaScript Articles
Let’s get started.
Why learn JavaScript?
Why should you learn JavaScript? Let’s start with the most obvious reason: employability.
According to HackerRank’s 2020 Developer report (PDF, 2.8 MB), JavaScript is the #1 sought-after language by hiring managers worldwide. It’s also the most commonly used language among professional developers, making it a must-have for your professional skill set.
In addition, many of the leading frameworks that power cutting-edge web development today, such as Node.js, jQuery and React, run on JavaScript. The language is essential for building dynamic and interactive websites, browsers, mobile apps, desktop programs and more. Knowing JavaScript won’t just boost your hiring potential; it’ll make you a better coder.
Skills covered in these tutorials:
- Variables & Objects
- Loops & Conditions
- Switch Statements
- Regular Expressions
- Chaining
- Boolean Operators
Ready to learn JavaScript and other skills needed to become a web developer? Check out Berkeley Coding Boot Camp and learn full-stack in 12 or 24 weeks.
Get Program Info
The JavaScript Language: Introduction
JavaScript is an object-oriented scripting language used most often in web development — although, as we mentioned earlier, it can be applied to other coding fields. As an object-oriented language (OOP), JavaScript depends on objects, or data structures that hold data and the applicable functions. OOPs are designed to allow coders to structure and modify programs quickly.
JavaScript is also a client-side language, which means that its source code passes through a client’s web browser instead of the webserver. This functionality cuts down on the amount of communication between a given page and its server and allows certain functions to run even after a web page has finished loading. Here’s a practical example — a JavaScript function might check on-page forms to ensure that all necessary fields are completed before sending any information to the server.
From a practical standpoint, JavaScript is often used in tandem with HTML and CSS to create dynamic, responsive web pages.
History of JavaScript
JavaScript is a language built with web development in mind. It was developed by Netscape, the creators of the leading browser of the time, Netscape Navigator, in collaboration with Sun Microsystems, the company that created Java, in 1995. In its earliest iterations, JavaScript was known as Mocha, Lichescript and ECMAScript, though none stuck quite as well as the name we know today.
JavaScript’s creators developed the language to address the new programming challenges posed by the internet. Before JavaScript, server-side languages were required to validate any data entered into a website, which made interactive features clunky at best. Making matters worse, most users at the time relied on dial-up connections, which slowed the communication between servers and web pages to a glacial pace.
JavaScript brought new utility and speed to early websites, and its usefulness only increased as internet connection speeds rose and the web expanded. Today, JavaScript is a fully fledged language that can facilitate complex interactions and build dynamic, engaging website features. Modern browsers like Google Chrome, Mozilla Firefox and Microsoft Edge are built to handle JavaScript quickly and accurately.
A brief note here — while JavaScript and Java share names and creators, the two languages don’t overlap all that much. While Java can be used to create web applications, it is more often used in game development and desktop app creation.
JavaScript Syntax
Here’s a quick JavaScript tutorial on the language’s syntax.
When you add code to your website, you’ll need to surround the instructions you plan to insert with HTML tags that indicate the programming language used — in this case, JavaScript. These tags will tell your browser that it needs to start processing JavaScript code.
Keep in mind that JavaScript ignores tabs, spaces and new lines; you can use all three freely to format code that is easy to read and understand.
Readability matters in JavaScript coding. For example, in languages like C++, Java and PHP, semicolons are essential to ending statements. However, in JavaScript, you can typically eliminate the semicolon by placing each statement on a separate line. If you want to put multiple statements on the same line, they must be separated by semicolons.
That said, it’s often considered good programming practice to use semicolons after each line, much as you would use a period at the end of a typed sentence — doing so helps future coders to understand what you created.
JavaScript is also case-sensitive. This means that “javascript” and “JavaScript” are not the same when it comes to processing your code. At the same time, you want to be clear and consistent, so it is not advised that you create multiple functions and variables with the same name but different capitalization. Stay on top of your use of uppercase and lowercase letters to ensure there are no mistakes!
By surrounding text in your script with characters such as /* or //, you can set it off as a comment. Your browser will not process comments as code, so you can leave notes that explain the purpose of specific lines within your code to other programmers.
JavaScript Variables and Types
JavaScript variables can hold several different data types, including numbers, strings (information entered as text, including numbers that are not calculated as an overall numerical value) and objects, which combine multiple strings and numbers into a single data structure.
Objects are the most critical data type in modern JavaScript because they can encompass multiple items into one comprehensive object. There are several other data types in JavaScript including null, for which the value is always zero, and undefined, a variable that is yet to be defined. JavaScript also includes a boolean data type, which can only have the value “true” or “false.”
Before using a JavaScript variable, you must declare it. JavaScript traditionally uses the keywords — “var” or “let” — to declare variables. Here is an example of declaring two variables:
var name;
var year;
This script would create two variables for “name,” and “year,” respectively. Each would have a value stored in them through a process called variable initialization. You could initialize the variable when you first create it, or you could do so later when using that variable. Here is an example:
name = ‘John Smith’;
year = 1982;
You could also declare and initialize your variable at the same time, as below:
let name = ‘John Smith’;
let year = 1982;
JavaScript is considered an “untyped” language. In some programming languages, you must declare the type of variable when it is created, specifying whether it will be a string, number, boolean, object or other data type. In JavaScript, the type of a variable can change as the program is executed; JavaScript handles this type of transition automatically.
JavaScript variables also have different scopes depending on where you define them. The language uses functions — subprograms that are designed to perform a particular task in the overall program. You can define variables to be used only within that function, or you can define variables to be used throughout an entire program.
It is worth noting that local, or in-function, variables take priority over global variables with the same name, so unless you want to overwrite a global variable specifically, you should track the different names you use for your variables to avoid unintended errors.
Like we mentioned earlier, when creating variable names, keep in mind that JavaScript is case-sensitive, so “year” and “Year” are two different variables. You can start your variable names with a letter or an underscore (_), but not a numeral. In addition, several reserved words are part of the JavaScript programming language (e.g., “false,” “debugger,” “continue”) and cannot be used as variable names.
Ready to learn more job-ready skills for a career in web development? Explore Berkeley Coding Boot Camp to start.
Get Program Info
What Are the Uses of JavaScript?
Now that we’ve discussed the basics of what JavaScript is and does, we can move on to its practical uses — namely, how you can apply the language in the professional world. Below, we’ve listed three professions that lean on JavaScript: front end web development, back end web development and game or mobile app development.
Front End Web Development
As we explained in our history section, JavaScript was designed with front end web development in mind. The language allows programmers to create dynamic pages and provide a responsive, engaging experience for website visitors. Developers who know JavaScript can also use libraries like Reveal.js, Swiper.js, Greensock.js and Anime.js, to enhance their images and animations.
JavaScript’s front end benefits aren’t limited to improving web page design, however. Programmers can also use JavaScript-based development frameworks such as React.js and Angular to produce complex, interactive web applications.
Back End Web Development
While JavaScript is used primarily for front end web development, you can also apply JavaScript frameworks and libraries on the back end if you have a runtime engine to support you.
A runtime engine provides the hardware and software infrastructure your codebase needs to execute programs in realtime. Essentially, an engine processes your JavaScript codebase into a form that a browser can readily interpret. Each browser has its own JavaScript engine — Chrome, for example, uses V8, while Firefox uses SpiderMonkey.
If you plan to use JavaScript in back end programming, you should also gain a baseline understanding of Node.js. Node.js is an open-source runtime environment that empowers coders to write JavaScript programs and execute them across operating systems such as OS X, Microsoft Windows and Linux.
If a back end programmer has JavaScript experience and the right runtime tools, they can quickly build complex web and software applications from the ground up.
Game and Mobile App Development
JavaScript can also come in handy for game developers.
As in back end development, game developers who want to code in JavaScript can use runtime engines to facilitate their work — two notable engines used in game development include Babylon.js and Phaser.js. JavaScript code can also be incorporated into larger game projects. Similarly, frameworks like React Native and NativeScript are designed to help developers build Android and iOS apps.
The tools you use in conjunction with your JavaScript skills will depend on your preferred medium, the type of game you want to write and your target players.
Which Careers Require Knowledge of JavaScript?
JavaScript is a foundational skill for those who want to build a career in programming. As we mentioned at the start of this article, the language is the #1 programming skill sought out by hiring managers globally and the most commonly known among professional developers.
Moreover, because development jobs are prevalent across a variety of industries, you could end up practicing your JavaScript skills in sectors such as finance, marketing, education, healthcare, government, manufacturing and more. Where you choose to plot your career path is up to you!
But that’s big-picture; let’s scale our view down to more immediate goals. Below, we’ve listed a few jobs that require JavaScript skills and could suit an entry-level professional.
JavaScript Developer
It should go without saying that JavaScript developers make use of JavaScript. In this position, you would be responsible for developing and implementing JavaScript code within the website’s front end. You could also build or update structures on the back end with runtime environments like Node.js. Your primary role would be developing and using your extensive familiarity with the language’s associated libraries and frameworks to build, improve and manage websites or applications.
Front End Developer
Front end developers are professional coders who use HTML, CSS and — of course — JavaScript to design, build and manage every aspect of a website that visitors interact with or see. This role requires programmers to balance their artistic sensibilities and programming savvy to create web-based products that are both beautiful and functional. These professionals can work independently as freelancers or contribute their expertise within a company’s programming team.
Application Developer
As an application developer, you would be responsible for developing and modifying existing source code for web and mobile applications. You may use JavaScript and other programming languages such as Java, C# or Python. As an app developer, you would be tasked with building applications from the ground up according to client specifications, as well as retooling or managing existing apps. Because building a fully fledged application usually requires a team, those who choose this professional track should have well-honed communication and collaboration skills.
Full Stack Developer
A full stack developer is a web developer with knowledge and training in all aspects of the development process. While a front end developer works with the user-facing part of a website and a back end developer works with the server-side technologies that drive a site, full stack developers have command over both. This command makes them well-suited to innovate new ways to link multiple aspects of a site and produce an integrated vision of a web project. JavaScript would be, of course, only one language in a full stack developer’s well-stocked toolbox.
How Long Does It Take to Learn JavaScript?
In short: it depends.
The time it takes you to learn JavaScript will depend heavily on whether you already have coding experience, what method you use to learn and how much time you can reasonably dedicate to studying.
If you are already a programmer or even a hobbyist who has some experience in HTML and CSS, you’ll probably be able to brush up on the fundamentals of JavaScript in a matter of weeks.
That said, if you’re a beginner with no coding experience to speak of, your turnaround time will almost certainly be longer. There are ways to speed up the process; for example, if you enroll in a full-time coding bootcamp, you could learn JavaScript — as well as several other languages and fundamental coding skills — in just three months. If you enroll in a part-time schedule, your timeline will extend to six months.
To summarize: even if you have little to no programming knowledge, you could gain a functional understanding in half a year if you enroll in a coding bootcamp.
If you prefer a more self-directed approach to your education, you can use online tutorials, books and coding tests to teach yourself JavaScript. In this case, your timeline would depend on how motivated you are about following a self-imposed curriculum. Some people might cover the fundamentals within a few months; others may require a year or more.
Of course, developing mastery is a lifelong project. There are always new frameworks and technologies on the horizon. Because JavaScript is an evolving language that continues to be on the cutting edge of web development tech, you will always actively be honing your skills.
What Else Should I Learn to Complement My JavaScript Skills?
In all likelihood, you won’t land a job with JavaScript skills alone. Instead, you’ll want to garner all of the skills you need to become a full stack web developer.
For example, programming languages like Python also drive many dynamic web applications and are especially useful for back end developers. Django is a popular open-source web application based on Python, which offers secure development and content management features.
Many of today’s popular websites run on SQL databases, and database manipulation can be a vital tool for both front end or back end developers.
Of course, front end frameworks and technologies are also critical. CSS, or Cascading Style Sheets, defines a website’s appearance, while the Bootstrap framework can empower you to achieve faster and more efficient results in web development.
If you are changing careers to move into tech, you don’t want to limit yourself too early. By having a sound foundation in both front end and back end development fundamentals, you will be in a great position to find a new job and explore potential career paths.
Want to get started? Check out Berkeley Coding Boot Camp! This intensive program focuses on full stack web development and can provide you with crucial JavaScript knowledge and other key skills you need to land an entry-level development job after three or six months of study. Contact us to learn more!
FAQs about JavaScript
JavaScript is one of the easiest programming languages to learn if you are just getting started. Because it is interpreted in your browser, you can test your code as you go along and see what works. However, if you’re just beginning, you may need to learn to think like a programmer. Enrolling in a formal JavaScript course can provide you the support, structure and guidance you need to adopt a coding mindset and succeed as a JavaScript developer.
Your timeline will vary depending on the level of knowledge you want to achieve and the level of experience you already have. If you are an experienced programmer, you could learn the fundamentals of JavaScript in a matter of weeks. However, if you are a beginner, you can expect your educational path to take several months. A coding bootcamp can help you achieve JavaScript fluency within three to six months.
JavaScript frameworks are becoming essential to modern web development. These collections of JavaScript code can make your development process faster and easier. Node.js, a runtime environment, is a crucial tool for back end development, while frameworks like React, Angular and Vue all have strong support in the JavaScript community. Learning core JavaScript skills is essential for success; you should always keep your eyes open for new and intriguing frameworks on the rise.
Many people compare JavaScript and Python, and they are two popular, widely used languages, especially in web development. Both have an assortment of libraries and frameworks, and both are good introductions for newer programmers. However, they are also different. Python is a full programming language, while JavaScript is a scripting language. Python is used more for server-side applications, while JavaScript is more popular for front end scripting. Both are great languages to have in your professional skill set.
Navigate JavaScript Articles
From basic knowledge to more advanced JavaScript coding techniques.
Get Program Info
Ready to learn more about Berkeley Coding Boot Camp in San Francisco? Contact an admissions advisor at (510) 306-1218.