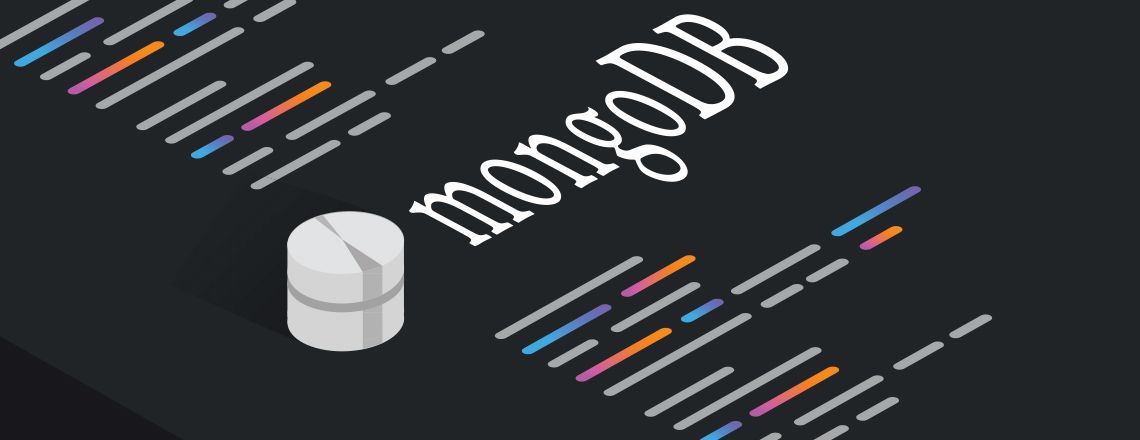
Node.js and MongoDB may seem complicated or time-consuming to master, but that is far from the truth. In this tutorial, we will show you how to use MongoDB and Node.js together in your web development projects.
You will also learn how to set up a server using Node.js and walk through establishing connections from Node.js to MongoDB databases. From there, you will learn how to select records using database calls and lastly, you will learn how to build and serve an HTML page with the data you retrieve.
Never Go It Alone
Both MongoDB and Node.js have dedicated communities that share their experiences and insights with fellow web developers. Connecting yourself to these communities is a powerful way to quickly learn new skills and develop better projects. If you’re a developer working in or around a tech hub like San Francisco, Node.js classes can provide a major added benefit and help you gain the knowledge and experience you need to start building better apps.
If you are serious about web development, ingratiating yourself into a community of fellow learners is the best way to network and learn.
Getting Started With MongoDB and Node.js
You will most likely begin by installing Node.js and MongoDB. Start by creating a Node.js server and install Node.js if you have not yet. You will then need a MongoDB database. If you want to create a database yourself, you can do so by using MongoLab, which provides free database hosting and other helpful paid services.
Your next step is to install MongoJS, the library used to connect Node.js with MongoDB databases. When you install Node.js, the software automatically installs NPM, making setup simple: open a terminal and select the directory where you want to put your Node.js server, then run npm install mongojs. Node.js’s automatic package manager automatically finishes the process from there.
Taking a Closer Look at MongoDB’s Server Code
Once you have completed the installations and setups, you can start writing the Node.js server by running the server on localhost. The first step is including the modules you will be using, including an HTTP module to create a server, as well as the MongoJS module you just installed:
After you include the modules you want to use, you must connect to the database. To do this, you need two vital components: a MongoDB connection URI and an array of collections you want to access in a MongoDB database.
MongoDB connection URIs are provided as the default whenever you create servers using MongoLab. As for the array of collections, these are just groups of MongoDB documents. In this case, you only need to access a single collection. However, you can have as many collections in an array as you want.
Next, you need to establish a connection. This is quite simple:
After you have called the createServer function, it will need another function for handling incoming requests. Your function will be called whenever browsers request data from your server. The function that handles requests, nicknamed requestHandler, uses a request variable and a response variable. The request variable represents browsers’ page requests, while the response variable is what browsers get in return for their requests. You can take a look at the requestHandler function below:
You’ll need to specify the format of your response to the user’s browser within the request handler. You can pick plain text, HTML or another format of your choice. Just make sure your request handler knows the format so it can manage incoming data.
Next, query your MongoDB database so that the information in it is available to you. Passing a JSON object to find your function lets you select a property you would like returned records to share. An iterable cursor containing all the information you need will return to the documents when you use the find function.
How to Engage Potential Problems
When using the find function, you’re working with a couple of variables: records and err. Err contains any data concerning errors, should they occur. Make sure to check whether any errors arose when you attempted your database query. If it went through without a hitch, you can move on, but if an error occurs, you will not see any available data and the remainder of your function will be useless. Log the issue and backtrack since there would be little reason to execute a whole function containing an error.
Once you have data from the cursor records, you must iterate the data and create an HTML string. The HTML string will provide something for the server to send to browsers. Make a variable to hold the string and iterate through your records. You need to build a string for each individual document and add it to your main string, like so:
After you are done iterating through your records, write a response with a quick while loop to generate an HTML string. Node.js gives you lots of options when displaying HTML, but the typical choice is to serve a static page that uses Express for its framework. However, generating a string is a fast, simple method for displaying basic HTML so you can try it out. Your HTML string will contain the entire page’s data, so you can call response.write to see that the client has received the information necessary for its operation. End the response, and the client should be able to load your page without a problem.
There you have it: the basics of creating an HTML server using Node.js and MongoDB. The last thing to do is tell your MongoDB server to listen at a specified port.
The code below will start a local server to access at port 8888. In your browser, this will show up as localhost:8888.
Working With MongoDB and Node.js in Future Projects
Using MongoDB and Node.js together can be much simpler than using many comparable web development technologies, since setting up the right error-handling and security can take more time and work than merely setting up and connecting your server.
By now, you can tell that setting up your own Node.js server connected to your own MongoDB database is a relatively easy task. If you are a web developer who wants to keep up with the best technologies, San Francisco Node.js classes could be the right fit for you, as they are a great opportunity for web developers to collaborate and learn.
*Please note, these articles are for educational purposes and the topics covered may not be representative of the curriculum covered in our boot camp. Explore our curriculum to see what you’ll learn in our program.
Get Program Info
Ready to learn more about Berkeley Coding Boot Camp in San Francisco? Contact an admissions advisor at (510) 306-1218.