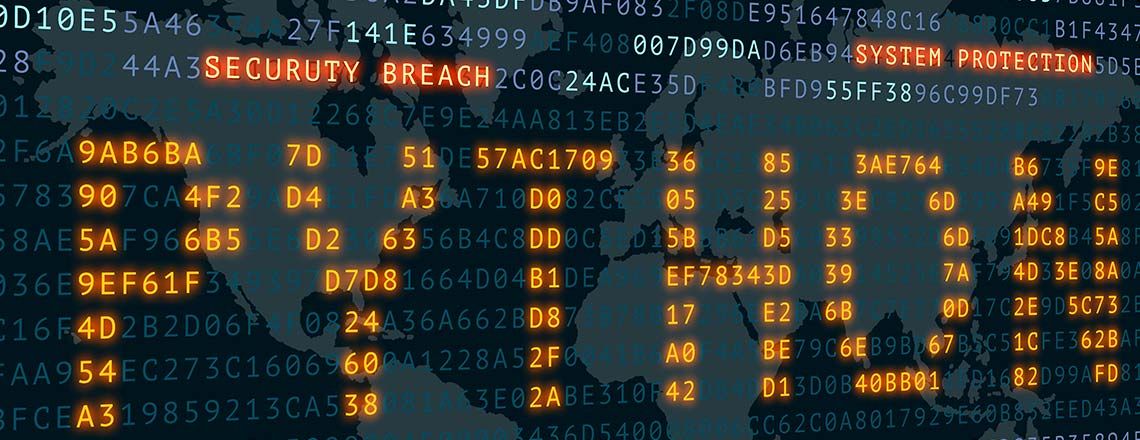
Python lists and tuples are similar to one another. If you’re considering enrolling in a Python bootcamp in San Francisco or another tech hub, start by learning the difference between the two sequence types.
Python List vs. Python Tuple
The biggest difference between Python lists and tuples is that a tuples are unchangeable and immutable. This means that its size and the items it contains cannot be modified. Let’s look at an example of what happens when you try to change items in your tuple:
TypeError: ‘tuple’ object does not support item
As you can see, running the above script returns an error message. However, if you have a mutable object, you may change it. Let’s look at the script for a Python list, a mutable object:
As you can see above, running the script for a Python list did not return an error message.
Python lists and tuples do share some similarities. For example, both are used to create a pointer list, which works with Python objects, also known as items. If you were to remove an object from a Python list, the item’s reference would be destroyed. However, the removed item is retrievable as long as your program still has references to it.
What Is a Python Tuple?
While tuples are not as popular as Python lists, they are still vital to working with data. If you have attended a data analytics bootcamp in the past, you may have been using tuples without even realizing it. For example, you may have been using tuples if:
- You worked with parameters and arguments.
- You used string formatting.
- You have returned at least two items using a function.
- You have iterated over a dictionary’s pairs.
When you run a program, you use thousands of tuples.
Empty Tuples vs. Empty Lists
An empty tuple will act like a singleton, which means that at all times, there will be just one tuple with a value of zero. If you create a tuple without anything in it, Python will point to a tuple that has already been pre-allocated, and the empty tuple will be assigned the address already in your memory. Python does this because it saves quite a bit of the computer’s memory. On the other hand, because lists can be modified, Python will not give it the same address already existing in the memory.
Optimizing Allocation With Small Tuples
Another way that Python keeps your computer’s memory free is by reusing an old tuple, which can also speed up the allocations. Rather than erasing a tuple that is no longer needed, the program moves it to another free list.
A free list contains 20 groups, each representing up to 2,000 Python tuples. The length of these tuples will be a value, n, between zero and 20. The first group always has a value of zero, which means that it will have a single, empty tuple. Let’s look at an example:
You can see that the code above uses the same identification number for groups a and b. This is because a tuple was briefly used once then destroyed, so its default replacement came from your free list. Using free tuples — instead of deleting them — can help speed up your computer system immensely.
Allocation Optimization and the Python List
Because a Python list can be modified, the optimization process is slightly different from that of a tuple. The Python list uses the free list for an empty object. If GC (Python’s automatic garbage collector) detects or deletes an empty Python list, it can still be used later on. Here is an example of what the process would look like in Python:
Resizing Your Python List
To further save computer memory, Python will not resize your list each time you remove or add an item. Instead, each list will have a certain number of slots without anything in them, determined by the current size of the list. You cannot see the slots, but they can still be utilized for a new item; if they are consumed, Python will allocate even more space for the items. Here is an example:
Over-allocating space gives the Python list more room for growth. While the extra allocation is slight, it is enough that amortized behavior will be able to take place. The pattern for the growth of a Python list is 0, 4, 8, 16, 25, 35, 46, 58, 72, 88, etc. Remember that new_allocated will not overflow with many items in it. The largest value is PY_SSIZE_T_MAX * (9 / 8) + 6, which will always fit size t.
To append one of your items to a list that has a length of eight, Python can resize the list to have 16 slots to allow a ninth item to be added to the list. However, the additional slots will not be visible until new items are added to the list.
Closing Thoughts
Whether or not you are planning on attending a Python bootcamp, this tutorial can help you understand how to utilize lists through optimization. Keep it on hand to revisit the practical examples of what you can do with a Python list above and read over the information to gain a full understanding of both the Python list and tuple.
*Please note, these articles are for educational purposes and the topics covered may not be representative of the curriculum covered in our boot camp. Explore our curriculum to see what you’ll learn in our program.
Get Program Info
Ready to learn more about Berkeley Data Analytics Bootcamp in San Francisco? Contact an admissions advisor at (510) 306-1218.